Python Typeerror: can't multiply sequence by non-int of type 'numpy.float64' [Solved]
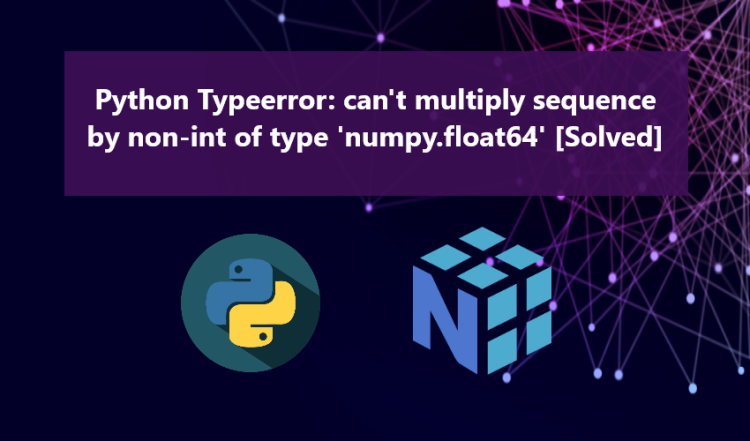
This article tackles into the resolution of a common error in the Python programming language, specifically the "Typeerror: can't multiply sequence by non-int of type 'numpy.float64'" error. Within this context, instances demonstrating this error will be presented, accompanied by an exploration of the underlying causes. Additionally, a corrected version of the example code will be supplied.
If you're encountering this Error in Python during your project's development phase, this article provides the solution you need to resolve it.
What is NumPy in Python?
Before we proceed to the primary objective of this tutorial, let's first grasp the concept of NumPy.
NumPy stands for "Numerical Python". It is a fundamental data structure by the NumPy Library of Python. It is a multidimensional, homogeneous, and memory-efficient container that allows you to store and manipulate large sets of numerical data. NumPy arrays are more efficient than Python's built-in lists for numerical computations because they are implemented in C and have specific optimizations for numerical operations.
Why "Typeerror: can't multiply sequence by non-int of type 'numpy.float64'" occurs in Python?
The "Typeerror: unable to multiply sequence by non-int of type 'numpy.float64'" commonly occurs when attempting a multiplication operation. This error signifies that the multiplication process is hindered due to the mismatch in data types between the two arrays, leading to the occurence of this specific error message.
This error message often materializes when utilizing NumPy Arrays for multiplication, and it is brought about by factors such as Improper Data Type Conversion, Incorrect Indexing, or Invalid Data Shape.
The mentioned Python Error shares a common cause and solution strategy when resolving the "Python TypeError: unable to multiply sequence by non-int of type float".
Examples
Here's an example Python script that simulates the Typeerror: can't multiply sequence by non-int of type 'numpy.float64'.
- import numpy
- #list of numbers
- arr1 = [1.0, 2.0, 3.0, 4.0]
- #numpy float64 value to multiply with the sequence (list) of number
- multiplyBy = numpy.float64(2.5)
- #performing the multiplication operation
- result = arr1 * multiplyBy
- print(result)
Solutions
In order to fixed the Python Typeerror that occured within the provided example simulation code, we can use the array() and multiply() functions.
Using the NumPy.array() Function:
Using the NumPy array() function, the sequenced list of numbers or integers will be compatible for multiplying with the Numpy float64().
- import numpy
- #list of numbers
- arr1 = numpy.array([1.0, 2.0, 3.0, 4.0])
- #numpy float64 value to multiply with the sequence (list) of number
- multiplyBy = numpy.float64(2.5)
- #performing the multiplication operation
- result = arr1 * multiplyBy
- print(result)
Using the NumPy multiply() Function
The NumPy multiply() will do the multiplication operation instead of using the asterisk (*) to prevent the error to occur.
- import numpy
- #list of numbers
- arr1 = [1.0, 2.0, 3.0, 4.0]
- #numpy float64 value to multiply with the sequence (list) of number
- multiplyBy = numpy.float64(2.5)
- #performing the multiplication operation
- result = numpy.multiply(arr1, multiplyBy)
- print(result)
This is how we can fix the TypeError: can't multiply sequence by non-int of type 'numpy.float64' based on the provided example Python simulation code.
Conclusion
The Python TypeError: can't multiply sequence by non-int of type 'numpy.float64' usually occurs due to incompatible data types in multiplication operations and can be solved using the provided solutions above.
I hope that this article will help you resolve the problems or errors you may be encountering during the development phase of your Python project. Feel free to explore more on this website for additional Tutorials, Free Source Codes, and articles on fixing bugs or errors that you might encounter in the future, such as the following:
- 737 views