How to fix the PHP "Illegal offset type" error
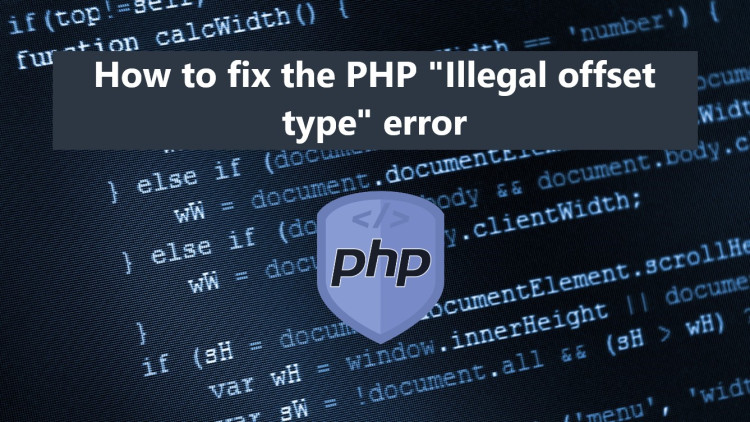
If you are currently facing a "Fatal error: Uncaught TypeError: Illegal offset type in ..." error in your PHP code or script, this article can help you to solve this kind of PHP error.
PHP's Illegal offset Type error occurs when you are trying to use a full object or array as the key of an array. PHP array keys can only be a string or integer type.
Example #1
Let's assume that we the following PHP code:
- <?php
- $myArray = [
- "key1" => "My Array Value 1",
- "key2" => "My Array Value 2",
- "key3" => "My Array Value 3"
- ];
- echo $myArray[$keys];
- ?>
In the PHP script above, we have an array with 3 items which have the key1, key2, and key3 keys. Each item has values which are "My Array Value 1" for "keys1", "My Array Value 2" for "keys2", and "My Array Value 3" for "keys3". The script will result in the following:
The error occurred because we are trying to echo or output something from the $myArray array with a full array as the key. To fix this Illegal offset type error, we should specify the exact array key to the $keys to get the value that we want and the value of the array with the specific key will serve as the key of the $myArray.
Here's the sample script that solves the Illegal offset type error on the code.
- <?php
- $myArray = [
- "key1" => "My Array Value 1",
- "key2" => "My Array Value 2",
- "key3" => "My Array Value 3"
- ];
- echo $myArray[$keys[0]]."<br/>";// output: My Array Value 1
- echo $myArray[$keys[1]]."<br/>";// output: My Array Value 2
- echo $myArray[$keys[2]];// output: My Array Value 3
- ?>
Example #2
The Illegal offset error might also occur if we unintentionally overwrite the value of a variable with an array. You must consider also to check the given variable to your array key has the right value that you intended to be returned.
Assuming that your PHP code is like the following.
- <?php
- $myArray = [
- "key1" => "My Array Value 1",
- "key2" => "My Array Value 2",
- "key3" => "My Array Value 3"
- ];
- $sampleVar = 'key1';
- // Unintended variable overwrite
- $sampleVar = [];
- echo $myArray[$sampleVar];
- ?>
Result
To solve this, we can either replace the variable name of the duplicate variable that causes the error with a unique variable or remove the duplicate variable if it is not in use. Check out the following fixed PHP script below.
- <?php
- $myArray = [
- "key1" => "My Array Value 1",
- "key2" => "My Array Value 2",
- "key3" => "My Array Value 3"
- ];
- $sampleVar = 'key1';
- // Replaced duplicate variable form $sampleVar into $sampleVar2
- $sampleVar2 = [];
- echo $myArray[$sampleVar];
- ?>
That's how you solve the Illegal offset. You can also leave a comment with any question or query about the PHP error you are currently and we'll try to help you find the solution.
That's it! I hope this How to Fix the PHP "Illegal offset type" error Tutorial will help you with what you are looking for.
Explore more on this website for more Tutorials and Free Source Codes.