Organizing MySQL Connection and CRUD in Visual Basic 2008 and MySQL Database
Submitted by janobe on Sunday, December 15, 2013 - 16:03.
In this tutorial I will show you how to organize MySQL Connection and CRUD by using a module. Module is one of the ways on how to organize your system, refining the code and it lessens your work. You can also access your code in different places.
Lets’ begin:
1. First create a MySQL Database named “userdb”.
3. Under the folder, create a Module and name it “concrud”.
4. After that, create a Connection of MySQL Database to Visual Basic 2008.
5. After creating a Connection. Do all the Declarations in Public.
6. Create the CRUD event.
7. Go back to Form and create a User Entry just like this.
8. Double click the Form and do this code.
9. Run your project.
You can download the complete Source Code and run it on your computer.
2. Open your Visual Basic 2008 and create a project. After that, in the solution explorer, right click and create “New Folder” , named “Modules”. I made this, to separate the Module and the Windows Form.
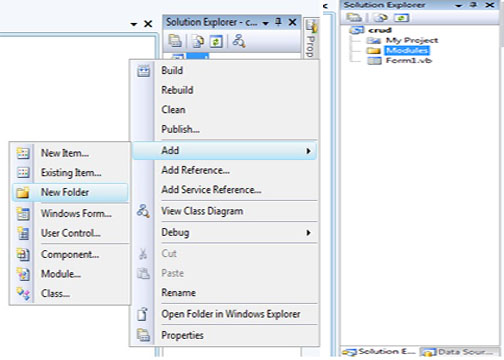
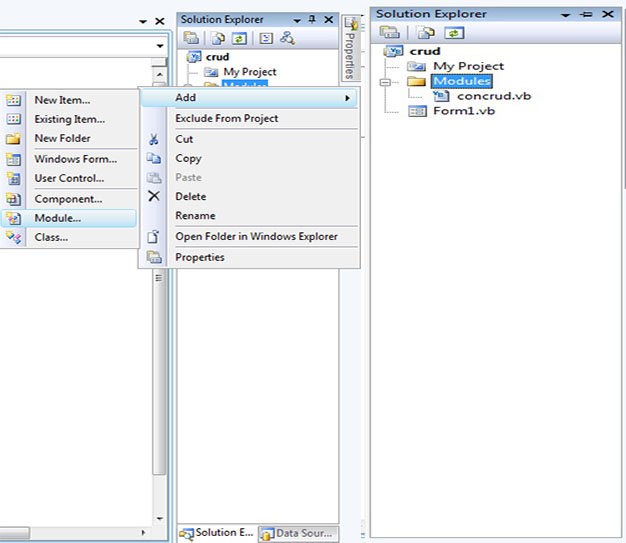
- Imports MySql.Data.MySqlClient
- Module concrud
- 'setting up your connection
- Public Function mysqlconnection() As MySqlConnection
- Return New MySqlConnection("server=localhost;user id=root;database=userdb")
- End Function
- Public con As MySqlConnection = mysqlconnection()
- End Modude
- ''declaring a string
- Public result As String
- ''declaring the class
- Public cmd As New MySqlCommand
- Public da As New MySqlDataAdapter
- Public dt As New DataTable
- ''for inserting the data to the database
- Public Sub create(ByVal sql As String)
- Try
- 'open the connection
- con.Open()
- 'holds the data
- With cmd
- .Connection = con
- .CommandText = sql
- 'execute the data
- result = cmd.ExecuteNonQuery
- If result = 0 Then
- Else
- End If
- End With
- Catch ex As Exception
- End Try
- End Sub
- 'for holding the data to retrieve.
- Public Sub reload(ByVal sql As String)
- Try
- dt = New DataTable
- con.Open()
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- Catch ex As Exception
- End Try
- da.Dispose()
- End Sub
- 'filling the table and retrieving the data to the datagridview from the database
- Public Sub filltable(ByVal dtg As DataGridView)
- Try
- con.Open()
- ''''''''
- '''''''''''''''fill table
- da.SelectCommand = cmd
- da.Fill(dt)
- dtg.DataSource = dt
- Catch ex As Exception
- End Try
- da.Dispose()
- End Sub
- 'updating the data from the database.
- Public Sub updates(ByVal sql As String)
- Try
- con.Open()
- With cmd
- .Connection = con
- .CommandText = sql
- result = cmd.ExecuteNonQuery
- If result = 0 Then
- Else
- End If
- End With
- Catch ex As Exception
- End Try
- End Sub
- 'deleting the data from the database
- Public Sub delete(ByVal sql As String)
- Try
- con.Open()
- With cmd
- .Connection = con
- .CommandText = sql
- result = cmd.ExecuteNonQuery
- If result = 0 Then
- Else
- End If
- End With
- Catch ex As Exception
- End Try
- End Sub
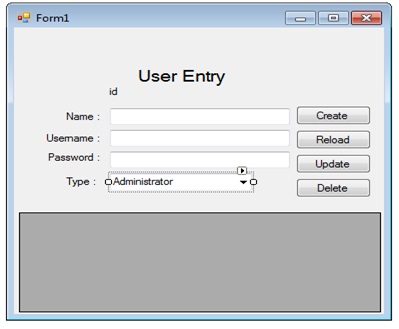
- Imports MySql.Data.MySqlClient
- Public Class Form1
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- ''"try" is for catching errors
- 'inserting of data.
- Try
- create("INSERT INTO users (`name`, `username`, `Pass`,`type`) VALUES ('" & TextBox1.Text & "','" _
- & TextBox2.Text & "','" & TextBox3.Text & "','" & ComboBox1.Text & "')")
- Catch ex As Exception
- End Try
- End Sub
- Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
- 'for retreiving the from the database
- Try
- reload("SELECT * FROM users")
- filltable(DataGridView1)
- Catch ex As Exception
- End Try
- End Sub
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- 'for updating data from the database..
- Try
- updates("UPDATE users SET `name` = '" & TextBox1.Text & "', `username` = '" & TextBox2.Text _
- & "', `Pass` = '" & TextBox3.Text & "',`type` = '" & ComboBox1.Text & "' where id = '" & lblid.Text & "'")
- Catch ex As Exception
- End Try
- End Sub
- Private Sub DataGridView1_DoubleClick(ByVal sender As Object, ByVal e As System.EventArgs) Handles DataGridView1.DoubleClick
- 'assigning data from the datagridview to the Label ,TextBox and ComboBox
- lblid.Text = DataGridView1.CurrentRow.Cells(0).Value
- TextBox1.Text = DataGridView1.CurrentRow.Cells(1).Value
- TextBox2.Text = DataGridView1.CurrentRow.Cells(2).Value
- TextBox3.Text = DataGridView1.CurrentRow.Cells(3).Value
- ComboBox1.Text = DataGridView1.CurrentRow.Cells(4).Value
- End Sub
- Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
- 'for deleting the data from the database
- Try
- delete("DELETE FROM users WHERE id = '" & lblid.Text & "'")
- Catch ex As Exception
- End Try
- End Sub
- End Class
Add new comment
- 279 views