PHP - Simple Login with md5
Submitted by razormist on Tuesday, May 7, 2019 - 22:21.
In this tutorial we will create a Simple Login with md5 using PHP. This code can protect the user login information by encrypting the password value. The code use a special php built-in function md5() a very strong encrypting tool that protect the user password by just passing the string in order to ultimately encrypt into a powerful password.
We will be using PHP as a scripting language and interpreter that is used primarily on any webserver including xamp, wamp, etc. It is being use to any famous websites and it has a modern technology that can easily be use by the next generation.
home.php
logout.php
There you have it we successfully created a Simple Login with md5 using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_md5, after that click Import then locate the database file inside the folder of the application then click ok.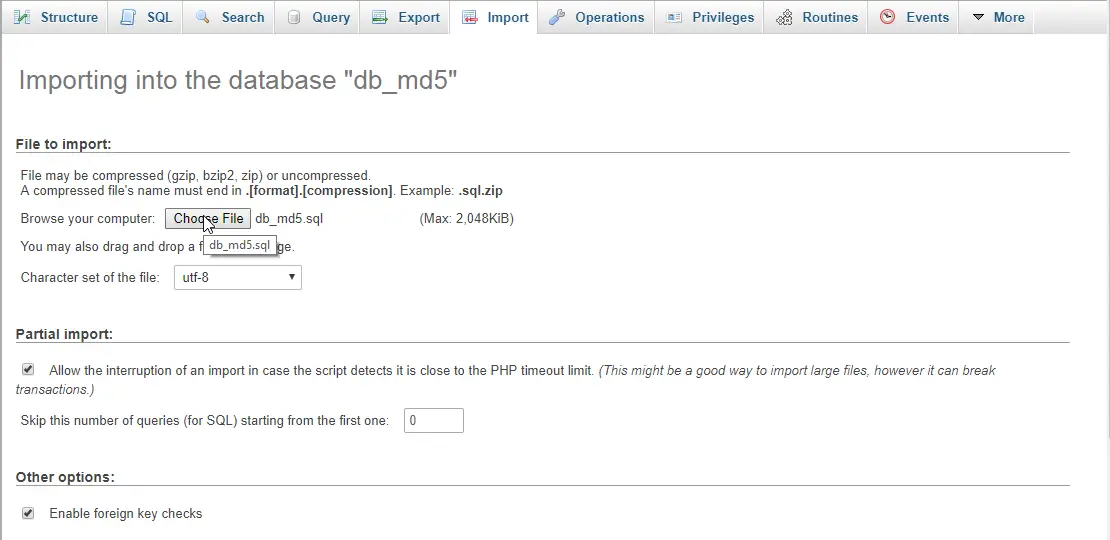
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as shown below. index.php- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Login with md5</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3">
- <h5>DEFAULT USER</h4>
- <h6>Username: admin</h6>
- <h6>Password: admin</h6>
- </div>
- <div class="col-md-6">
- <form method="POST" >
- <div class="form-group">
- <label>Username</label>
- <input type="text" name="username" maxlength="50" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" name="password" maxlength="12" class="form-control" required="required"/>
- </div>
- <?php include 'login.php'?>
- <center><button class="btn btn-primary" name="login"><span class="glyphicon glyphicon-log-in"></span> Login</button></center>
- </form>
- </div>
- </div>
- </body>
- </html>
- <!DOCTYPE html>
- <?php
- require_once 'conn.php';
- }
- ?>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Login with md5</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <?php
- $query=mysqli_query($conn, "SELECT * FROM `user` WHERE `user_id`='$_SESSION[user_id]'") or die(mysqli_error());
- ?>
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <h1>WELCOME</h1>
- <h3 class="text-primary"><?php echo $fetch['name']?></h3>
- <a href="logout.php" class="btn btn-primary"><span class="glyphicon glyphicon-log-out"></span> Logout</a>
- </div>
- </div>
- </body>
- </html>
Creating the Main Function
This code contains the minor function of the application. This code will allow the user to login with a md5 encrypted protection. To do this just kindly write these block of codes inside the text editor, then save it as shown below. login.php- <?php
- require_once 'conn.php';
- $username = $_POST['username'];
- $query = mysqli_query($conn, "SELECT * FROM `user` WHERE `username` = '$username' && `password` = '$password'") or die(mysqli_error());
- if($rows > 0){
- $_SESSION['user_id'] = $fetch['user_id'];
- }else{
- echo "<center><label class='text-danger'>Invalid username or password!</label></center>";
- }
- }
- ?>
- <?php
- ?>