PHP - Display Videos from YouTube Channel
Submitted by razormist on Wednesday, September 5, 2018 - 15:48.
In this tutorial we will create a Display Videos from YouTube Channel using PHP. This code can be use for storing of list of data. PHP is a server-side scripting language designed primarily for web development. Using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user friendly environment. So Let's do the coding...
Then, after the project is created go to Credentials and generate your key here.
There you have it we successfully created Display Videos from YouTube Channel using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into you text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Get Videos from YouTube Channel</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button type="button" class="btn btn-primary" id="search"><span class="glyphicon glyphicon-search"></span> Get Video</button>
- <div id="result"></div>
- </div>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script type="text/javascript">
- $(document).ready(function(){
- $('#search').on('click', function(){
- $('#result').load('get_video.php');
- });
- });
- </script>
- </body>
- </html>
Getting the Youtube API key
In order to get your api key, first you need to login your google accounts. Then visit this site https://console.developers.google.com/apis/dashboard After that create a project here.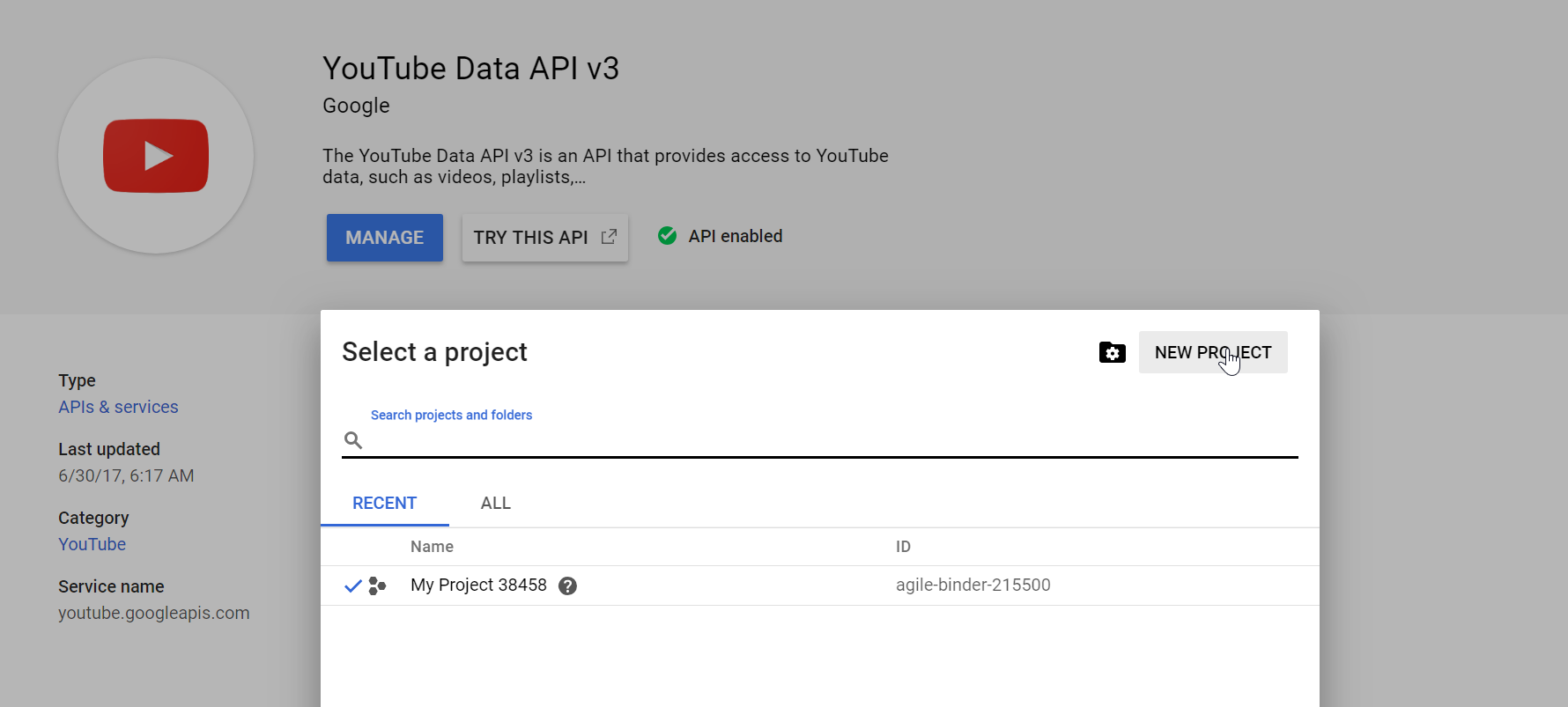
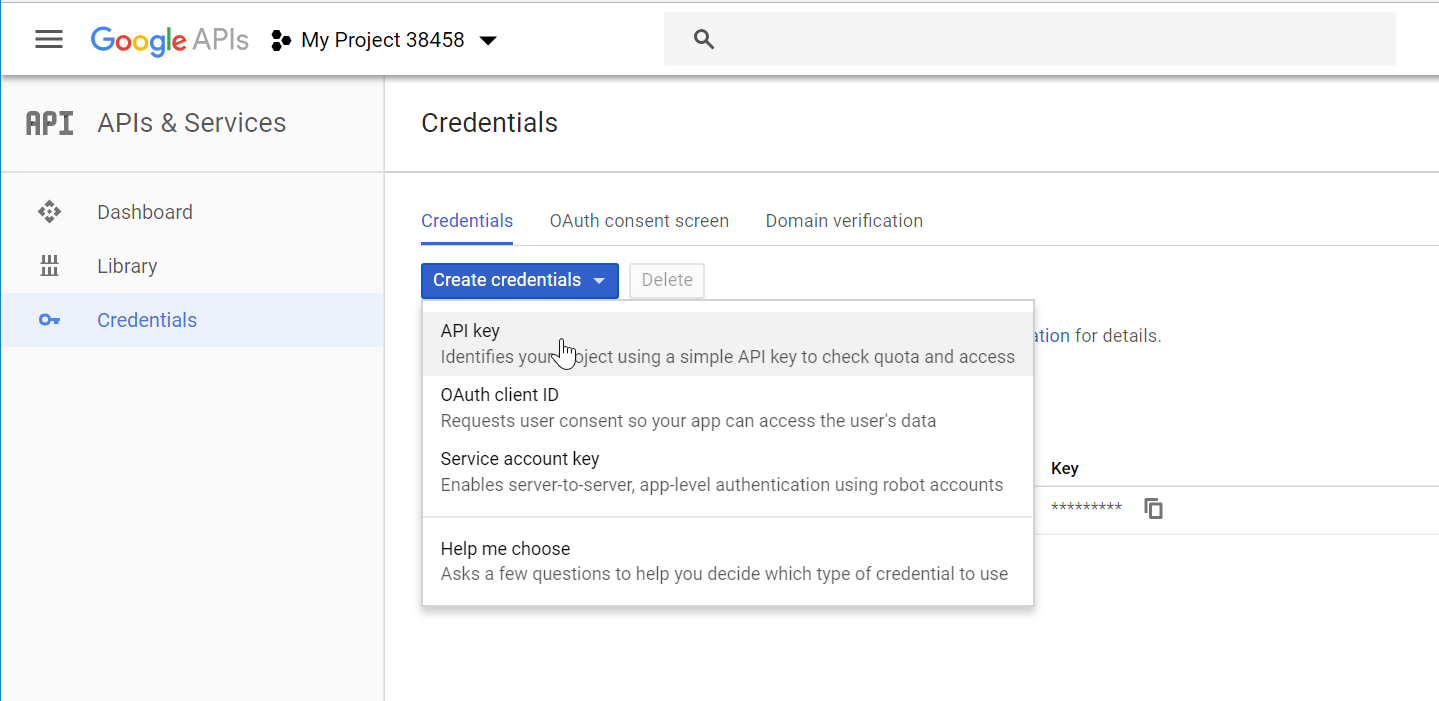
Setting the Keys
This section hold the api key for the youtube videos. To do this copy and write the codes inside the text editor then save it as youtube_key.php- <?php
- $api_key = "YOUR API KEY';
- $video_id = 'UC28FIDM6e_MVxFq4AQXcUxQ';
- ?>
Creating the Main Function
This code contains the main function of the application. This code will get the list of the youtube videos channel. To do this just kindly copy and write these block of codes inside the text editor, then save it as get_video.php.- <?php
- require_once 'youtube_key.php';
- $videos = json_decode(file_get_contents('https://www.googleapis.com/youtube/v3/search?order=date&part=snippet&channelId='.$video_id.'&maxResults=50&key='.$api_key.''));
- foreach($videos->items as $item){
- ?>
- <div style="float:left; width:250px; margin:20px;">
- <center><h5><?php echo $item->snippet->title ?></h5></center>
- <br />
- <center><iframe width="230" height="180" src="https://www.youtube.com/embed/<?php echo $item->id->videoId ?>" frameborder="0" allowfullscreen></iframe></center>
- </div>
- <?php
- }
- }
- ?>