PHP - OOP Inserting Multiple Rows in One Form using AJAX/jQuery
Submitted by nurhodelta_17 on Wednesday, November 1, 2017 - 13:16.
Getting Started
Please take note that CSS and jQuery used in this tutorial are hosted so you need internet connection for them to work.Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as testing. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `member` (
- `memberid` INT(11) NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(50) NOT NULL,
- `lastname` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`memberid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
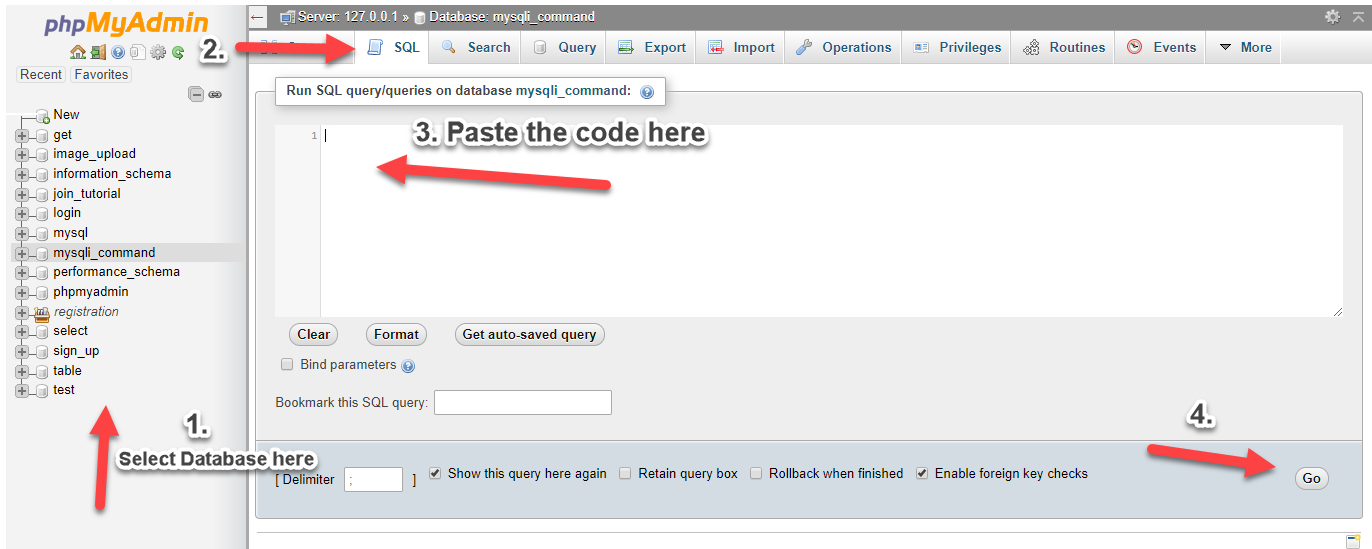
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- $conn = new mysqli("localhost", "root", "", "testing");
- if ($conn->connect_error) {
- }
- ?>
index.php
This is our index that contains our sample table and our add form.- <!DOCTYPE html>
- <html>
- <head>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div class="col-md-8 col-md-offset-2">
- </div>
- </div>
- <div class="row">
- <div class="col-md-8 col-md-offset-2">
- <form id="addForm">
- <hr>
- <div class="row">
- <div class="col-md-2">
- </div>
- <div class="col-md-10">
- <input type="text" name="firstname[]" class="form-control">
- </div>
- </div>
- <div class="row">
- <div class="col-md-2">
- </div>
- <div class="col-md-10">
- <input type="text" name="lastname[]" class="form-control">
- </div>
- </div>
- <hr>
- <div class="row">
- <div class="col-md-12">
- </div>
- </div>
- </form>
- </div>
- </div>
- </div>
- </body>
- </html>
custom.js
This contains our AJAX and jQuery codes.- $(document).ready(function(){
- showTable();
- $('#newbutton').click(function(){
- $('#newrow').slideDown();
- var newrow = '<hr>';
- newrow = '<div class="row">';
- newrow += '<div class="col-md-2"><label style="position:relative; top:7px;">Firstname:</label></div>';
- newrow += '<div class="col-md-10"><input type="text" name="firstname[]" class="form-control"></div>';
- newrow += '</div>';
- newrow += '<div style="height:10px;"></div>';
- newrow += '<div class="row">';
- newrow += '<div class="col-md-2"><label style="position:relative; top:7px;">Lastname:</label></div>';
- newrow += '<div class="col-md-10"><input type="text" name="lastname[]" class="form-control"></div>';
- newrow += '</div>';
- newrow += '<hr>';
- $('#newrow').append(newrow);
- });
- $('#save').click(function(){
- var addForm = $('#addForm').serialize();
- $.ajax({
- type: 'POST',
- url: 'add.php',
- data: addForm,
- success:function(data){
- if(data==''){
- showTable();
- $('#addForm')[0].reset();
- $('#newrow').slideUp();
- $('#newrow').empty();
- }
- else{
- showTable();
- $('#addForm')[0].reset();
- $('#newrow').slideUp();
- $('#newrow').empty();
- alert('Rows with empty field are not added');
- }
- }
- });
- });
- });
- function showTable(){
- $.ajax({
- type: 'POST',
- url: 'fetch.php',
- data:{
- fetch: 1,
- },
- success:function(data){
- $('#table').html(data);
- }
- });
- }
fetch.php
This is our code in fetching our sample table.- <?php
- include('conn.php');
- ?>
- <table class="table table-bordered table-striped">
- <thead>
- <th>Firstname</th>
- <th>Lastname</th>
- </thead>
- <tbody>
- <?php
- include('conn.php');
- $query=$conn->query("select * from member");
- while($row=$query->fetch_array()){
- ?>
- <tr>
- <td><?php echo $row['firstname']; ?></td>
- <td><?php echo $row['lastname']; ?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- <?php
- }
- ?>
add.php
Lastly, this is our code in adding new row into our database.- <?php
- include('conn.php');
- $firstname = $_POST["firstname"];
- $lastname = $_POST["lastname"];
- if($firstname_clean != '' && $lastname_clean != ''){
- $conn->query("insert into member (firstname, lastname) values ('".$firstname_clean."', '".$lastname_clean."')");
- }
- else{
- echo "1";
- }
- }
- }
- ?>