Voter's ID Finder for Supreme Student Government using Visual basic.Net
Submitted by joken on Monday, September 23, 2013 - 17:30.
This time, I'm going to show you how to create a "Voter's ID finder" for Supreme Student Government. The system also has a load of all data from the database and you can also search for specific student by using the quick search and display the result to the datagrid.
To start building this project, you need to add two textbox, three buttons, three label and a datagridview and last a timer. Then name the button1 as “btnload” and the Text property to “Load Data”, next the button2 name it as “bntsearch” and chande the Text property to “Search” and last the button3 name is as “btnexit” and chage the Text property to “Exit”. And retain all properties for the rest of objects available. Then the final output of this program after designing should look like as shown
This time, we need to add functionality to our program. To do this double click our main form, then under public class add the following code:
The new added code is a function, and this function will hold our two technology name as “Provider” and “Data Source” and will always return a new Connection. And our database name is “votedb” and it is stored in the “bin” folder.
Next, inside the form load, add the following code:
Then we will add functionality to our “btnload” button, to do this double click this button and add the following code.
Next, to double click “btnsearch”, and the following code:
It displays the Type: Lastname or Course and textbox and so that it can allow user input for searching specific student.
This time, we will add functionality for our quick search option. To do this, add the following code:
To display the time, double click the “timer” and add the following code:
And finally double click the “btnexit” button, and add the following code:
This simply terminate the application.
This time, you can now test your program by pressing “F5”.
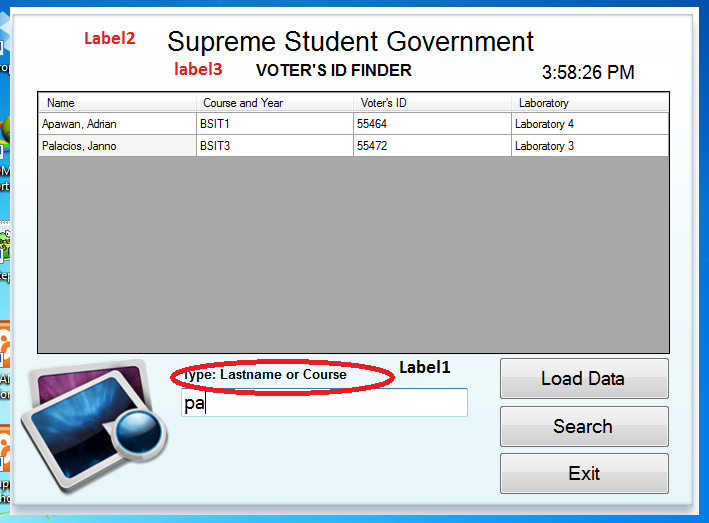
- Private Function GetConnection() As OleDb.OleDbConnection
- Return New OleDb.OleDbConnection("Provider=Microsoft.ACE.OLEDB.12.0;" & "Data Source=" & Application.StartupPath & "\votedb.accdb")
- End Function
- 'will hide the Type: Lastname or Course and textbox and start the timer for time
- Label1.Hide()
- TextBox1.Hide()
- Timer1.Start()
- Private Sub btnload_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnload.Click
- 'declare dt as new datatable, this will hold later the value from data adapter
- Dim dt As New DataTable
- 'declare conn as connection and it will now a new connection because it is equal to Getconnection Function
- Dim conn As OleDb.OleDbConnection = GetConnection()
- Try
- 'this is our query to display all the values from the table
- Dim sql As String = "SELECT Name, Course_yr, voteid, Laboratory FROM votersid"
- 'bind sql statement and connection and pass the result to da
- Dim da As OleDb.OleDbDataAdapter = New OleDb.OleDbDataAdapter(sql, conn)
- 'it fills the value from da to datatable named dt
- da.Fill(dt)
- 'fill the datagridview by getting fron dt
- DataGridView1.DataSource = dt
- Finally
- 'close connection
- End Try
- End Sub
- Label1.Show()
- TextBox1.Show()
- With TextBox1.Text
- Focus()
- End With
- Private Sub TextBox1_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles TextBox1.TextChanged
- Dim dt As New DataTable
- Dim conn As OleDb.OleDbConnection = GetConnection()
- Try
- Dim sql As String = "SELECT Name, Course_yr, voteid, Laboratory FROM votersid where Name Like '%" & TextBox1.Text & "%'or Course_yr like'%" & TextBox1.Text & "%'"
- Dim da As OleDb.OleDbDataAdapter = New OleDb.OleDbDataAdapter(sql, conn)
- da.Fill(dt)
- DataGridView1.DataSource = dt
- Finally
- conn.Dispose()
- End Try
- End Sub
- 'it gets the current time and this is based on local machine
- TextBox2.Text = TimeOfDay
Add new comment
- 100 views