No Layout in Java (Absolute Positioning)
Submitted by donbermoy on Friday, December 5, 2014 - 08:15.
This tutorial will teach you how to provide a java swing program without a layout. We will use the keyword null here in the setLayout method and we will do the Absolute Positioning using the setBounds method.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of noLayout.java.
2. Import javax.swing.* package library import javax.swing.*; //used to access JFrame, JLabel, and JTextField class.
3. Now, we will have a User Interface of having the LookAndFeelDecorated UI and set it to true.
We will initialize variables in our Main, variable frame as JFrame, label1 and label2 as JLabel, and textField1 and textField2 as JTextField.
4. To set the layout without a layout, we will use the keyword null here in the setLayout method of the frame.
Now, we will have an absolute positioning of our components and we will use the setBounds method. The setBounds method specify the x-position, y-position, width of the components, and height of the components respectively. Here, we will use the setBounds method of the two labels and two textfields and make sure that they don't overlap with each other.
5. Add then the components using the add method.
Lastly, set its size, visibility to true, and have its close operation.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; //used to access JFrame, JLabel, and JTextField class
- frame.getContentPane().setLayout(null);
- label1.setBounds(20, 20, 200, 40);
- label2.setBounds(20, 60, 200, 40);
- textField1.setBounds(125, 20, 200, 40);
- textField2.setBounds(125, 65, 200, 40);
- frame.getContentPane().add(label1);
- frame.getContentPane().add(textField1);
- frame.getContentPane().add(label2);
- frame.getContentPane().add(textField2);
- frame.setSize(400, 200);
- frame.setVisible(true);
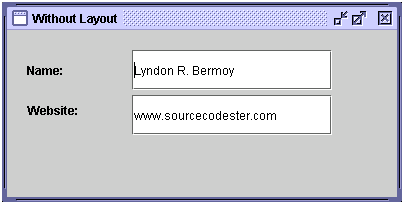
- import javax.swing.*; //used to access JFrame, JLabel, and JTextField class
- public class noLayout{
- label1.setBounds(20, 20, 200, 40);
- label2.setBounds(20, 60, 200, 40);
- textField1.setBounds(125, 20, 200, 40);
- textField2.setBounds(125, 65, 200, 40);
- frame.getContentPane().setLayout(null);
- frame.getContentPane().add(label1);
- frame.getContentPane().add(textField1);
- frame.getContentPane().add(label2);
- frame.getContentPane().add(textField2);
- frame.setSize(400, 200);
- frame.setVisible(true);
- }
- }