Compute Number of Days to Months and Days in Java GUI
Submitted by donbermoy on Monday, May 19, 2014 - 14:17.
This tutorial will teach you how to compute a given number of days to months and days using Java GUI. For example we input a number of days of 65, the equivalent months and days is 2 months and 5 days.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of monthsDays.java.
2. Import javax.swing package. Hence we will use a GUI (Graphical User Interface) here like the inputting the number of days.
3. Initialize your variables in your Main, variable numInput as string and make it as an inputdialogbox. Variable months and days as integer for parsing the inputted number of days, and variable m and d as integer also for getting the equivalent months and days.
4. Compute the equivalent months and days by dividing the months by 30 and get the remainder of 30 in days.
5. Lastly, display the output of the program using JOptionPane.showMessageDialog.
Output:
Here's the full code of this tutorial:
Hope this program helps! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*;
- int days, months;
- String numInput;
- int d,m;
- d = days%30;
- m = months/30;
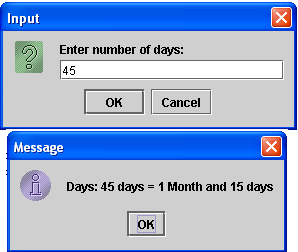
- import javax.swing.*;
- public class monthsDays{
- int days, months;
- String numInput;
- int d,m;
- d = days%30;
- m = months/30;
- }
- }
Add new comment
- 97 views