How to Find Sum of Odd Factors from a Given Number in Python
In this tutorial, we will program 'How to Find the Sum of Odd Factors of a Given Number in Python.' We will learn how to find and calculate the sum of all odd factors based on the given number. The objective is to automatically calculate the sum of the odd factors of the given number. I will provide a sample program to demonstrate the actual coding process in this tutorial.
This topic is very easy to understand. Just follow the instructions I provide, and you will be able to do it yourself with ease. The program I will show you covers the basics of programming for calculating the sum of all odd factors. I will do my best to provide you with a simple method for displaying the sum of these odd factors. So, let's start with the coding.
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import math
- def sumofoddFactors( n ):
- res = 1
- while n % 2 == 0:
- n = n // 2
- for i in range(3, int(math.sqrt(n) + 1)):
- count = 0
- curr_sum = 1
- curr_term = 1
- while n % i == 0:
- count+=1
- n = n // i
- curr_term *= i
- curr_sum += curr_term
- res *= curr_sum
- if n >= 2:
- res *= (1 + n)
- return res
- ret = False
- while True:
- print("\n================= Find Sum of Odd Factors from a Given Number =================\n\n")
- n = int(input("Enter a number: "))
- print("The sum of odd number is: ",sumofoddFactors(n))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This Python script calculates the sum of odd factors of a given number. It first removes even factors, then computes the sum of factors for each odd divisor up to the square root of the number. The program repeatedly prompts the user to input a number, displays the sum of its odd factors, and asks if they want to try again. The loop continues until the user chooses to exit.
Output:
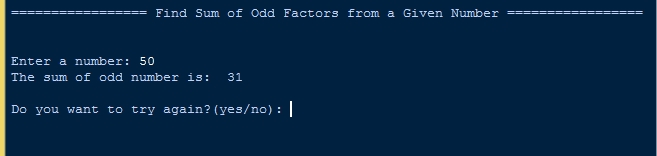
There you have it we successfully created How to Find Sum of Odd Factors from a Given Number in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 76 views