MDAS Calculation Using a Class
Submitted by janobe on Sunday, January 5, 2014 - 20:34.
In this tutorial I will teach you how to create the MDAS calculation within the class using Visual Basic 2008. This will help you organize your code and minimize bunch of codes in your Windows Form. MDAS stands for Multiplication, Division, Addition and Subtraction.
Let’s begin:
Open Visual Basic 2008, create a Project named “MDAS” and set up your Form just like this.
Add a new file which is a class and name it “calculate”. After that, do this code inside the class.
Go back to the Design Views, double click the Form and create a sub procedure for the clicking event of the radio button.
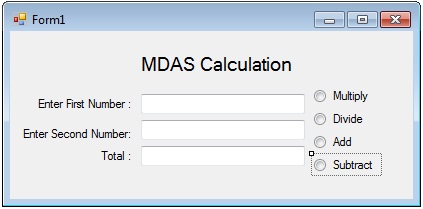
- Public Class calculate
- 'declare a private variable as a double
- Private _num1 As Double
- Private _num2 As Double
- Private _total As Double
- 'set a readonly property for the total of the MDAS
- ReadOnly Property total() As Double
- Get
- Return _total 'return the total
- End Get
- End Property
- 'create a property for a private variable so that you can access it
- Property num1() As Double
- Get
- Return _num1 'return the first value
- End Get
- Set(ByVal value As Double)
- _num1 = value 'set the first value
- End Set
- End Property
- Property num2() As Double
- Get
- Return _num2
- End Get
- Set(ByVal value As Double)
- _num2 = value
- End Set
- End Property
- 'create the sub procedures of the MDAS
- Public Sub multiply()
- 'formula of multiplication
- _total = num1 * num2
- End Sub
- Public Sub divide()
- 'formula of division
- _total = num1 / num2
- End Sub
- Public Sub add()
- 'formula of addition
- _total = num1 + num2
- End Sub
- Public Sub subtract()
- 'formula of subtraction
- _total = num1 - num2
- End Sub
- End Class
- Public Class Form1
- 'create a sub procedure for the events of clicking the radio button that handles all of it.
- Private Sub RadioButton_Click(ByVal sender As Object, ByVal e As System.EventArgs) _
- Handles Radio_Subtaction.Click, Radio_Multiplication.Click, Radio_Division.Click, Radio_Addition.Click
- 'call a constructor method and return to cal as an instance of a class
- Dim cal As calculate = New calculate()
- 'declaring the string variable represent as a textbox
- Dim txtnum1 As String = TextBox1.Text
- Dim txtnum2 As String = TextBox2.Text
- 'declaring the double variable
- Dim dbl_val1 As Double
- Dim dbl_val2 As Double
- 'convert the string to double
- dbl_val1 = Double.Parse(txtnum1)
- dbl_val2 = Double.Parse(txtnum2)
- 'get the value of the converted variable
- 'to pass it into the variable in the class
- cal.num1 = dbl_val1
- cal.num2 = dbl_val2
- 'the condition is, if the radiobutton is clicked,
- 'the operation of MDAS executes.
- If Radio_Multiplication.Checked Then
- 'result:
- cal.multiply() 'call a subname in a class for multiplying
- ElseIf Radio_Division.Checked Then
- 'result:
- cal.divide() 'call a subname in a class for dividing
- ElseIf Radio_Addition.Checked Then
- 'result:
- cal.add() 'call a subname in a class for adding
- ElseIf Radio_Subtaction.Checked Then
- 'result:
- cal.subtract() 'call a subname in a class for subtracting
- End If
- Else
- 'the result is:
- 'if the textbox is empty or has a string value
- TextBox3.Text = "Enter a number"
- Return
- End If
- 'put the result of the MDAS to a textbox.
- TextBox3.Text = cal.total()
- End Sub
- End Class
Add new comment
- 1086 views