Sending E-mail using VB.NET
Submitted by donbermoy on Wednesday, February 5, 2014 - 15:22.
Hi! In this tutorial, i will introduce some kind advanced VB.NET Tutorial which is Sending an E-mail.
VB.Net allows sending e-mails from your application. The System.Net.Mail namespace contains classes used for sending e-mails to a Simple Mail Transfer Protocol (SMTP) server for delivery.
In this tutorial, let us create a simple application that would send an e-mail. Take the following steps:
Next, type the following code:
The SmtpClient class allows applications to send e-mail by using the Simple Mail Transfer Protocol (SMTP).
Following are some commonly used properties of the SmtpClient class:
Property Description
And the result of this when clicking the button will be sent to your e-mail. Example is this image below that it was sent to my e-mail address.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
- Add three labels, three text boxes and a button control in the form.
- Change the text properties of the labels to - 'From', 'To:' and 'Message:' respectively.
- Change the name properties of the texts to txtFrom, txtTo and txtMessage respectively.
- Change the text property of the button control to 'Send'
- Add the following code in the code editor.
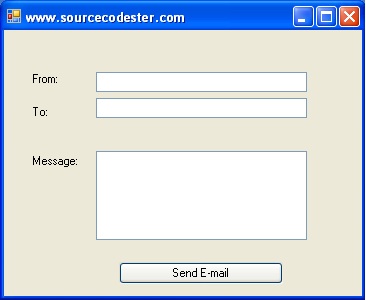
- Imports System.Net.Mail
- Public Class Form1
- Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs) Handles MyBase.Load
- ' Set the caption bar text of the form.
- Me.Text = "www.sourcecodester.com"
- End Sub
- Private Sub Button1_Click(ByVal sender As Object, ByVal e As EventArgs) Handles Button1.Click
- Try
- Dim Smtp_Server As New SmtpClient
- Dim e_mail As New MailMessage()
- Smtp_Server.UseDefaultCredentials = False
- Smtp_Server.Port = 587
- Smtp_Server.EnableSsl = True
- Smtp_Server.Host = "smtp.gmail.com"
- e_mail = New MailMessage()
- e_mail.From = New MailAddress(txtFrom.Text)
- e_mail.To.Add(txtTo.Text)
- e_mail.Subject = "Email Sending"
- e_mail.IsBodyHtml = False
- e_mail.Body = txtMessage.Text
- Smtp_Server.Send(e_mail)
- MsgBox("Mail Sent")
- Catch error_t As Exception
- MsgBox(error_t.ToString)
- End Try
- End Sub
- End Class
- ClientCertificates Specifies which certificates should be used to establish the Secure Sockets Layer (SSL) connection.
- Credentials Gets or sets the credentials used to authenticate the sender.
- EnableSsl Specifies whether the SmtpClient uses Secure Sockets Layer (SSL) to encrypt the connection.
- Host Gets or sets the name or IP address of the host used for SMTP transactions.
- Port Gets or sets the port used for SMTP transactions.
- Timeout Gets or sets a value that specifies the amount of time after which a synchronous Send call times out.
- UseDefaultCredentials Gets or sets a Boolean value that controls whether the DefaultCredentials are sent with requests.
- You must specify the SMTP host server that you use to send e-mail. The Host and Port properties will be different for different host server. We will be using gmail server.
- You need to give the Credentials for authentication, if required by the SMTP server
- You should also provide the email address of the sender and the e-mail address or addresses of the recipients using the MailMessage.From and MailMessage.To properties, respectively.
- You should also specify the message content using the MailMessage.Body property
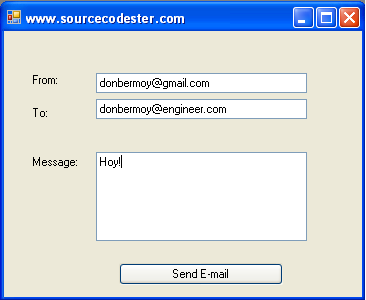
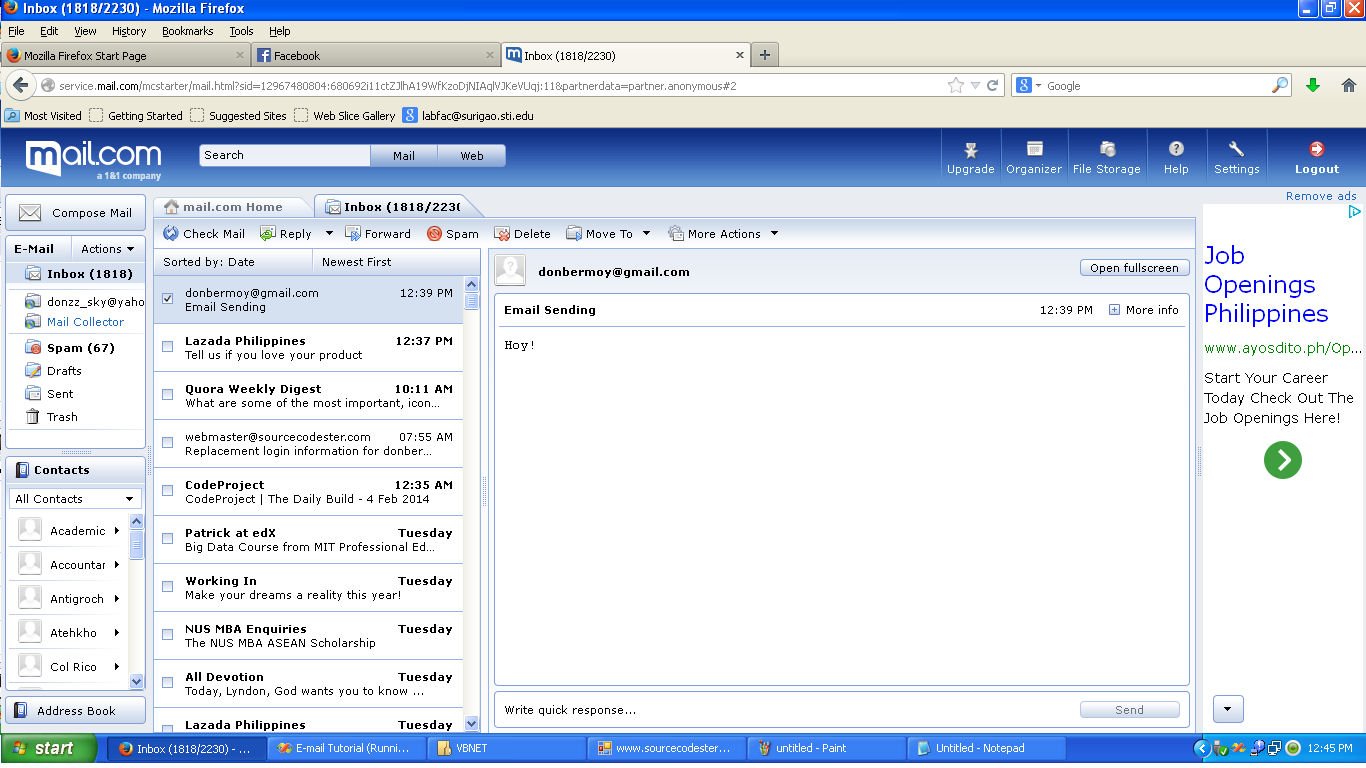
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz