Easy Picture Viewer
Submitted by Dr Dave on Tuesday, December 10, 2013 - 06:54.
This program was created in Microsoft Visual Basic 2010 Express to demonstrate how to use OpenFileDialog control.
Create a new Windows Form Application project and add a PictureBox, three Buttons, and the OpenFileDialog controls to the form and arrange as in the picture below.
Set control properties:
The code for setting the file filter is
If desired, you can have each file type on a separate line in the drop down list by changing the code as follows
In order to select more than one file at the time, the following code is needed
To call the File Dialog, start by clearing the text in the initial filename and use the ShowDialog Function to generate the File Dialog window. When the File Dialog window closes it generates a return code. Typically, the only error that you should be concerned about is if the Cancel button was selected or the window is closed without selecting a file. To catch this, use the If-Then statement below to exit the subroutine if the Cancel return code is generated
The list of filenames are recovered with the following
Start the file counter to zero and load the first picture into the PictureBox Image using the following
To move to the next picture, increment the file counter and enable the Previous Button. Check to see if you have reached the last file in the list; if so, disable the Next Button. Load the next picture into the PictureBox.
To return to the previous picture, decrement the file counter and enable the Next Button. If you have reached the first file, disable the Previous Button.
The complete code
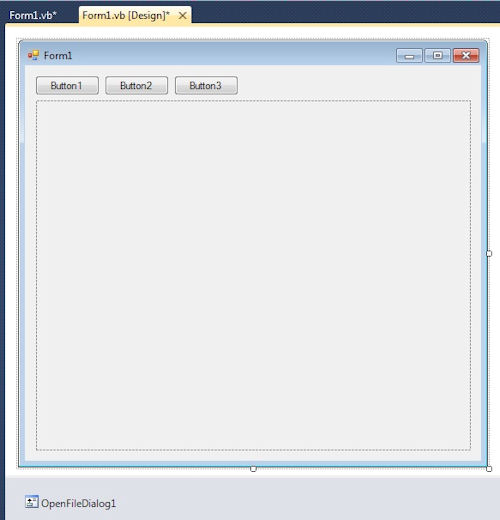
Control | Property | Value |
PictureBox1 | Anchor | Top, Bottom, Left, Right |
Size Mode | Zoom | |
Button1 | Name | btnOpenFiles |
Text | OopenFiles | |
Button2 | Name | btnPrev |
Enabled | False | |
Text | Previous | |
Button3 | Name | btnNext |
Enabled | False | |
Text | Next | |
OpenFileDialog1 | No Changes |
OpenFileDialog1.Filter = "Pictures Files|*.jpg;*.gif;*.tif;*.png;*.bmp|All files|*.*"
The filter text is in the form of text descriptor and search string separated by a pipe (|). The search string is usually asterisk (*.) followed by the file extension desired. The code above will result in the drop down list shown in the picture below.
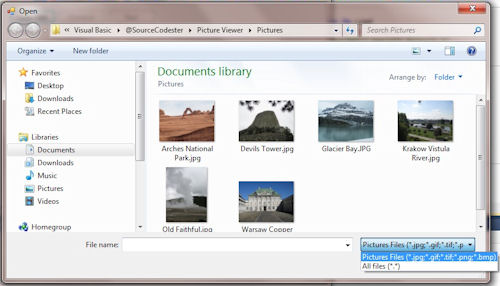
OpenFileDialog1.Filter = "JPEG|*.jpg|GIF|*.gif|TIFF|*.tif|PNG|*.png|BMP|*.bmp|All files|*.*"
The drop down list now looks like this
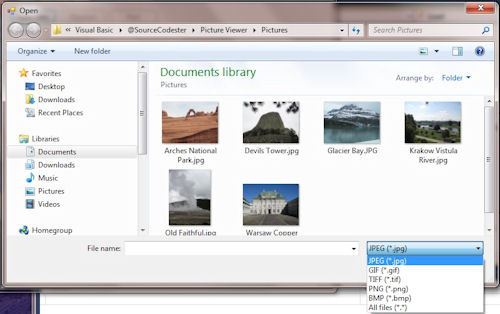
OpenFileDialog1.Multiselect = True
I put these two lines of code in the Form1_Load event but can be set in the design properties if desired.
- Private Sub Form1_Load(sender As Object, e As System.EventArgs) Handles Me.Load
- OpenFileDialog1.Filter = "Pictures Files|*.jpg;*.gif;*.tif;*.png;*.bmp|All files|*.*"
- OpenFileDialog1.Multiselect = True
- End Sub
- OpenFileDialog1.FileName = ""
- If OpenFileDialog1.ShowDialog() = vbCancel Then Exit Sub
Filenames = OpenFileDialog1.FileNames
Where Filenames is an undimensioned array Dim Filenames() As String
The array is zero-based so the list of files will be indexed from 0 to N. So to find out how many files were selected, you can either use Filenames.length which will have a value of N+1 or you can us UBound(Filenames) which will have a value of N. For this program, UBound will be used.
If more than one filename was selected, the Next Button is enabled otherwise it will be disabled to prevent an error. The Previous Button will be disabled to also prevent an error.
- btnPrev.Enabled = False
- FilenamesCounter = 0
- PictureBox1.Image = Image.FromFile(Filenames(0))
- FilenamesCounter = FilenamesCounter + 1
- btnPrev.Enabled = True
- PictureBox1.Image = Image.FromFile(Filenames(FilenamesCounter))
- FilenamesCounter = FilenamesCounter - 1
- btnNext.Enabled = True
- If FilenamesCounter = 0 Then btnPrev.Enabled = False
- PictureBox1.Image = Image.FromFile(Filenames(FilenamesCounter))
- Dim Filenames() As String
- Dim FilenamesCounter As Integer
- Private Sub Form1_Load(sender As Object, e As System.EventArgs) Handles Me.Load
- OpenFileDialog1.Filter = "Pictures Files|*.jpg;*.gif;*.tif;*.png;*.bmp|All files|*.*"
- 'OpenFileDialog1.Filter = "JPEG|*.jpg|GIF|*.gif|TIFF|*.tif|PNG|*.png|BMP|*.bmp|All files|*.*"
- OpenFileDialog1.Multiselect = True
- End Sub
- Private Sub btnOpenFiles_Click(sender As System.Object, e As System.EventArgs) Handles btnOpenFiles.Click
- OpenFileDialog1.FileName = ""
- If OpenFileDialog1.ShowDialog() = vbCancel Then Exit Sub 'Cancel
- Filenames = OpenFileDialog1.FileNames
- btnPrev.Enabled = False
- FilenamesCounter = 0
- PictureBox1.Image = Image.FromFile(Filenames(0))
- End Sub
- Private Sub btnPrev_Click(sender As System.Object, e As System.EventArgs) Handles btnPrev.Click
- PictureBox1.Image.Dispose()
- FilenamesCounter = FilenamesCounter - 1
- btnNext.Enabled = True
- If FilenamesCounter = 0 Then btnPrev.Enabled = False
- PictureBox1.Image = Image.FromFile(Filenames(FilenamesCounter))
- End Sub
- Private Sub btnNext_Click(sender As System.Object, e As System.EventArgs) Handles btnNext.Click
- PictureBox1.Image.Dispose()
- FilenamesCounter = FilenamesCounter + 1
- btnPrev.Enabled = True
- PictureBox1.Image = Image.FromFile(Filenames(FilenamesCounter))
- End Sub
Add new comment
- 61 views