Get Computer Drive using C#
Submitted by donbermoy on Thursday, July 17, 2014 - 19:22.
This is a simple tutorial entitled "Get Computer Drive using C#". Sometimes we have require to get the drives of our computer, but this tutorial gets the list of available drives in ListView control.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, add only a ListView named ListView1 to display all the available drives in your computer. You must design your layout like this:
3. Now, put this code in your code view.
We have imported Imports
The syntax above indicates that whatever the DriveType is such as a Removable Drive or a Fixed Drive, it will all display in the ListView.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
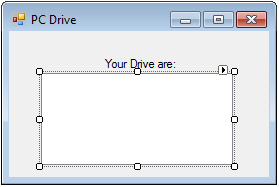
- using System.IO;
- public class Form1
- {
- private void Form1_Load(System.Object sender, System.EventArgs e)
- {
- object getDrive = default(object);
- foreach (object tempLoopVar_getDrive in Environment.GetLogicalDrives)
- {
- getDrive = tempLoopVar_getDrive;
- if (InfoDrive.DriveType == DriveType.Removable | InfoDrive.DriveType == DriveType.Fixed)
- {
- ListView1.Items.Add(getDrive);
- }
- }
- }
- }
using System.IO;
to get the IO devices such as the drive. This library contains the DriveInfo method and the DriveType method.
We also initialized variable getDrive to be used in the For Each Loop for foreach (object tempLoopVar_getDrive in Environment.GetLogicalDrives) Method. This method returns an array of string containing the names of the logical drives on the current computer.
- if (InfoDrive.DriveType == DriveType.Removable | InfoDrive.DriveType == DriveType.Fixed)
- {
- ListView1.Items.Add(getDrive);
- }
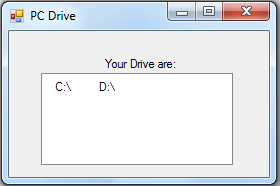
Add new comment
- 31 views